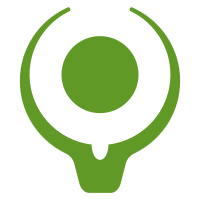
- changed username field on the login form to the type "text" as "name" is no valid type which causes problems with some extensions (password managers)
67 lines
2.4 KiB
JavaScript
67 lines
2.4 KiB
JavaScript
$(document).ready(function() {
|
|
// Show element counter for tables
|
|
$('[data-toggle="tooltip"]').tooltip();
|
|
var rowCountDomainAlias = $('#domainaliastable >tbody >#data').length;
|
|
var rowCountDomain = $('#domaintable >tbody >#data').length;
|
|
var rowCountMailbox = $('#mailboxtable >tbody >#data').length;
|
|
var rowCountAlias = $('#aliastable >tbody >#data').length;
|
|
var rowCountResource = $('#resourcetable >tbody >#data').length;
|
|
$("#numRowsDomainAlias").text(rowCountDomainAlias);
|
|
$("#numRowsDomain").text(rowCountDomain);
|
|
$("#numRowsMailbox").text(rowCountMailbox);
|
|
$("#numRowsAlias").text(rowCountAlias);
|
|
$("#numRowsResource").text(rowCountResource);
|
|
|
|
// Filter table function
|
|
$.fn.extend({
|
|
filterTable: function(){
|
|
return this.each(function(){
|
|
$(this).on('keyup', function(e){
|
|
var $this = $(this),
|
|
search = $this.val().toLowerCase(),
|
|
target = $this.attr('data-filters'),
|
|
$target = $(target),
|
|
$rows = $target.find('tbody #data');
|
|
$target.find('tbody .filterTable_no_results').remove();
|
|
if(search == '') {
|
|
$target.find('tbody #no-data').show();
|
|
$rows.show();
|
|
} else {
|
|
$target.find('tbody #no-data').hide();
|
|
$rows.each(function(){
|
|
var $this = $(this);
|
|
$this.text().toLowerCase().indexOf(search) === -1 ? $this.hide() : $this.show();
|
|
})
|
|
if($target.find('tbody #data:visible').size() === 0) {
|
|
var col_count = $target.find('#data').first().find('td').size();
|
|
var no_results = $('<tr class="filterTable_no_results"><td colspan="100%">-</td></tr>')
|
|
$target.find('tbody').prepend(no_results);
|
|
}
|
|
}
|
|
});
|
|
});
|
|
}
|
|
});
|
|
$('[data-action="filter"]').filterTable();
|
|
$('.container').on('click', '.panel-heading span.filter', function(e){
|
|
var $this = $(this),
|
|
$panel = $this.parents('.panel');
|
|
$panel.find('.panel-body').slideToggle("fast");
|
|
if($this.css('display') != 'none') {
|
|
$panel.find('.panel-body input').focus();
|
|
}
|
|
});
|
|
$('.container').on('click', '.panel-heading .panel-title', function(e){
|
|
var $this = $(this),
|
|
$panel = $this.parents('.panel');
|
|
$panel.find('.table-responsive').slideToggle("fast");
|
|
});
|
|
$('.panel-heading .panel-title').addClass('clickable');
|
|
$('.panel .table-responsive').each(function() {
|
|
if ($(this).height() > 550) {
|
|
// If one is too large initially hide all
|
|
$('.panel .table-responsive').slideUp("fast");
|
|
}
|
|
})
|
|
});
|